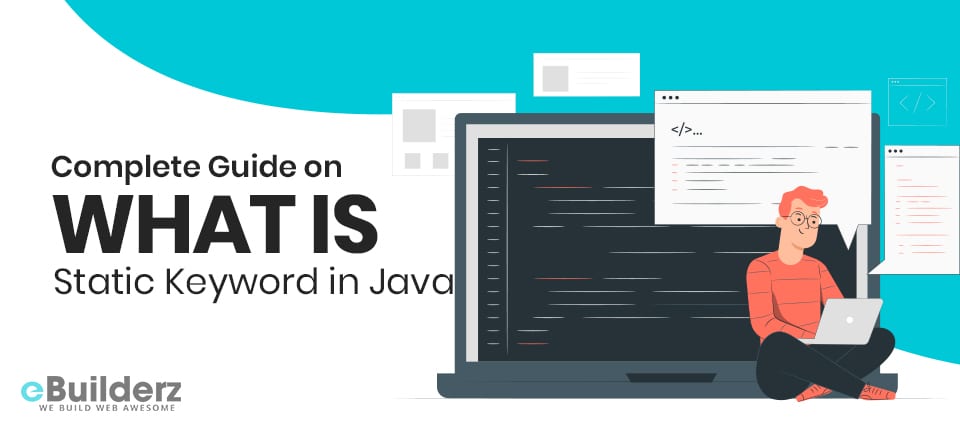
Keywords are reserved words in Java that can’t be used as identifiers. In Java, we have 57 keywords in total. Among those keywords is one called Static. In this article, we are going to look at how to apply static in various aspects of programming when using Java.
Here are some of the topics that we are going to cover in this article:
- Static keyword in Java
- How to apply Static keyword
- Static Block
- Static Variable
- Static Method
- Static Classes
Static Keyword in Java
Programmers use static keywords in Java to manage memory. Programmers use it with methods, variables, nested classes, and blocks. With a static keyword, you can share the method of a class or the same variable. You can use it with the same method for every instance of a class or a constant variable. Generally, the main method of a class is labeled static.
You need to precede a static member with the keyword static if you create it. That is the block, variable, method, or nested class. You don’t need any object reference when a member is declared as static since you can access it before creating objects of its class. Being a non-access modifier in Java programming language, this is what you can use the static keyword for:
- Static Method
- Static Classes
- Static Block
- Static Variable
With an example, we will explain in detail each of these methods to help you understand better.
Also, you can read Significance of Keywords in SEO: A Beginner’s Guide
How to Apply Static Keyword
Before we show you how to apply static keywords in Java, we will first show you how the Java programming language is enhanced with static blocks.
Static Block
When you load the class, and you want to do your computation to initialize your static variables, all you need to do is declare a static block to be executed once. The diagram below will help you understand the use of Static Block in a Java program.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
|
// Java program to demonstrate the use of static blocks
import java.util.*;
public class BlockExample{
// static variable
static int j = 10;
static int n;
// static block
static {
System.out.println(“Static block initialized.”);
n = j * 8;
}
public static void main(String[] args)
{
System.out.println(“Inside main method”);
System.out.println(“Value of j : “+j);
System.out.println(“Value of n : “+n);
}
}
|
I believe that you have understood what a static block is and how to use it. Next, we will be looking at what static variables are and how they help.
Output:
1
2
3
4
|
Static block initialized
Inside main method
Value of j:10
Value of n : 80
|
Static Variable
Declaring a variable as static means that at the class level, a single copy of the variable is created and divided among all objects. In other words, global variables are static variables. The same static valuables are shared with all the instances of the class. You can also create static variables at the class-level only.
Read More: How to study for a programming test?
The image below will help us understand what this means:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
|
// Java program demonstrate execution of static blocks and variables
import java.util.*;
public class VariableExample
{
// static variable
static int j = n();
// static block
static {
System.out.println(“Inside the static block”);
}
// static method
static int n() {
System.out.println(“from n “);
return 20;
}
// static method(main !!)
public static void main(String[] args)
{
System.out.println(“Value of j : “+j);
System.out.println(“Inside main method”);
}
}
|
Output:As you can see, by executing the program above, a static block and the variables will be executed in order.
1
2
3
4
|
from n
Inside the static block
Value of j: 20
Inside main method
|
We trust that we have explained what static variables are clearly. Let’s now explain in detail the meaning of nested classes and Static methods in the Java Static keyword.
Static Methods
A static method refers to a method that is declared with the static keyword. The main ( ) method is a good example of a static method. The following restrictions will be experienced when methods are declared as static:
- They can only call other static methods directly.
- Static data is directly accessed.
Here is a diagram that will help you understand what static methods are more clearly:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
|
// java program to demonstrate restriction on static methods
public class StaticMethodExample
{
// static variable
static int j = 100;
// instance variable
int n = 200;
// static method
static void a()
{
a = 200;
System.out.println(“Print from a”);
// Cannot make a static reference to the non-static field b
n = 100; // compilation error
// Cannot make a static reference to the
// non-static method a2() from the type Test
a2(); // compilation error
// Cannot use super in a static context
System.out.println(super.j); // compiler error
}
// instance method
void a2()
{
System.out.println(“Inside a2”);
}
public static void main(String[] args)
{
// main method
}
}
|
Static ClassAs you can see from these examples, restrictions are imposed on static methods. The diagram also shows how you can use the super keyword in the static context. That’s it. We hope that you have understood what Static Methods are. Let us now move to nested classes.
The only time a class static is when it’s a nested class. You don’t need a referral from the Outer class to use a nested static class. This means that non-static members of the Outer class can’t be accessed by a static class.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
public class NestedExample{
private static String str= “Edureka”
//Static class
static class MyNestedClass{
//non-static method
public void disp(){
System.out.println(str);
}
}
public static void main(String args[]){
NestedExample.MyNestedClass obj = new NestedExample.MyNestedClass();
obj.disp();
}
|
Checkout quick video review on Static Java Keyword
Source: Telusko
Conclusion
We have shared with you the various applications of the Static Keyword in Java. We believe that you have understood clearly what the Static keyword is and how it is used in Java. We also have other articles about Java on our blog if you need more information.
Here are a few more topics that you shouldn’t miss:
Complete Guide: How to Become A Python Developer?
How to Fix “Unable to get Local Issuer SSL Certificate” Error?
Freshbooks Review [2020]: Best Accounting Software For Small Businesses
Like this post? Don’t forget to share
No comment yet, add your voice below!